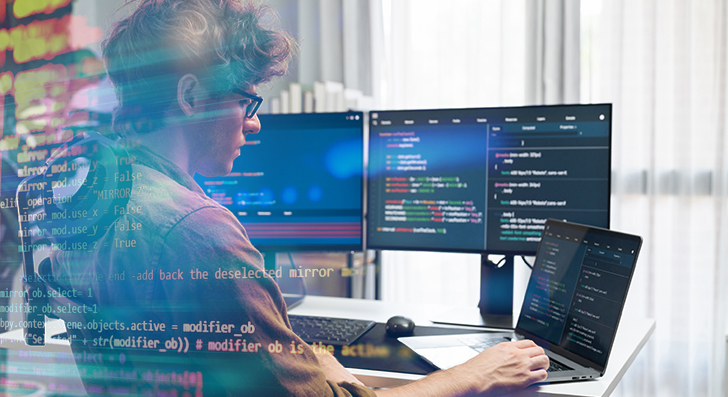
Scalability suggests your software can cope with expansion—a lot more customers, more facts, plus much more targeted traffic—with out breaking. As a developer, making with scalability in mind saves time and worry later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your prepare from the beginning. A lot of applications are unsuccessful after they mature quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you need to Consider early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent elements. Each module or support can scale By itself without the need of affecting The entire process.
Also, give thought to your database from day a single. Will it need to deal with 1,000,000 end users or simply just 100? Choose the correct sort—relational or NoSQL—determined by how your facts will develop. Program for sharding, indexing, and backups early, Even though you don’t want them nevertheless.
A different vital point is to prevent hardcoding assumptions. Don’t compose code that only performs below existing problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design patterns that support scaling, like message queues or event-driven methods. These assist your app handle much more requests devoid of having overloaded.
After you Establish with scalability in your mind, you are not just getting ready for success—you're decreasing long term complications. A effectively-planned system is less complicated to keep up, adapt, and expand. It’s greater to organize early than to rebuild later.
Use the proper Databases
Selecting the correct database is usually a key Element of building scalable purposes. Not all databases are built exactly the same, and using the Erroneous you can sluggish you down as well as result in failures as your app grows.
Get started by knowledge your info. Can it be hugely structured, like rows inside a table? If Certainly, a relational database like PostgreSQL or MySQL is a superb healthy. They're strong with interactions, transactions, and consistency. In addition they assistance scaling methods like examine replicas, indexing, and partitioning to deal with extra targeted traffic and data.
If the information is much more flexible—like consumer exercise logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your examine and publish styles. Have you been executing lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases which can handle large produce throughput, or simply occasion-based mostly facts storage units like Apache Kafka (for short-term info streams).
It’s also sensible to Assume ahead. You may not need to have State-of-the-art scaling features now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Avoid unnecessary joins. Normalize or denormalize your information according to your accessibility patterns. And often check database efficiency while you expand.
To put it briefly, the ideal databases will depend on your application’s framework, pace wants, And the way you count on it to develop. Consider time to pick sensibly—it’ll conserve lots of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every small hold off provides up. Badly prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting clear, straightforward code. Steer clear of repeating logic and take away just about anything unwanted. Don’t select the most complex Alternative if an easy 1 is effective. Maintain your functions shorter, targeted, and easy to check. Use profiling equipment to locate bottlenecks—sites in which your code requires far too extended to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These generally slow points down greater than the code alone. Make certain Each individual query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively pick unique fields. Use indexes to speed up lookups. And prevent doing too many joins, Primarily across massive tables.
For those who recognize the exact same data getting requested over and over, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In place of updating a row one by one, update them in groups. This cuts down on overhead and would make your application extra efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 records may well crash whenever they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These measures support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more traffic. If everything goes through one server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. As an alternative to one particular server carrying out all of the function, the load balancer routes users to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused quickly. When people request the same information and facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly obtain.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, increases speed, and would make your app far more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Alongside one another, they help your app take care of more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Tools
To create scalable apps, you would like instruments that permit your app develop very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t really have to buy hardware or guess long term capability. When site visitors will increase, it is possible to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and security tools. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it straightforward to move your application amongst environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy simply, and Get better immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and assist you to remain centered on building, not repairing.
Watch Every thing
In case you don’t observe your application, you received’t know when things go Improper. Checking allows the thing is how your app is executing, place challenges early, and make much website better choices as your application grows. It’s a critical part of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just observe your servers—monitor your app as well. Keep watch over how long it will take for consumers to load webpages, how often problems take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for significant complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or even a support goes down, you ought to get notified right away. This assists you repair issues speedy, normally in advance of end users even observe.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and see a spike in faults or slowdowns, it is possible to roll it back again before it results in true injury.
As your application grows, website traffic and info increase. Without the need of monitoring, you’ll miss indications of difficulty until it’s far too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app responsible and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking stressed. Commence small, Feel major, and Develop sensible.